Add Admob Interstitial Ads To Your Sketchware Project Using AIDE
1) Export your Project from Sketchware. The project will be in the format of zip file.Extract it.Check whether any files are missing.In my case the build.gradle file was missing.So I copied the build.gradle file of my previous project and pasted it .
Note : After pasting , change the application id in the build.gradle file to the package name of the project in which you want to add Ads
Skip the Above steps if your project file contains build.gradle
2) Open the project in AIDE . Navigate to build.gradle which is in the app directory
Add the following code
compile 'com.google.android.gms:play-services-ads:+'
3)Navigate to AndroidManifest.xml and add the following Code
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
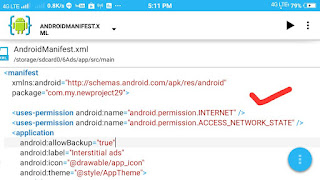
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
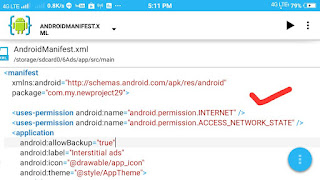
Also add the activity
<activity android:name="com.google.android.gms.ads.AdActivity"
android:configChanges="keyboard|keyboardHidden|orientation|screenLayout|uiMode|screenSize|smallestScreenSize"
android:theme="@android:style/Theme.Translucent" />
android:configChanges="keyboard|keyboardHidden|orientation|screenLayout|uiMode|screenSize|smallestScreenSize"
android:theme="@android:style/Theme.Translucent" />
4)Open MainActivity.java .Add the following Code.
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.AdRequest;
import com.google.android.gms.ads.InterstitialAd;
import com.google.android.gms.ads.AdListener;
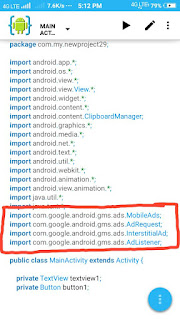
private InterstitialAd mInterstitialAd;
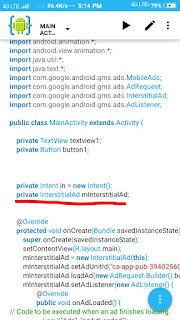
mInterstitialAd = new InterstitialAd(this);
mInterstitialAd.setAdUnitId("ca-app-pub-3940256099942544/1033173712");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
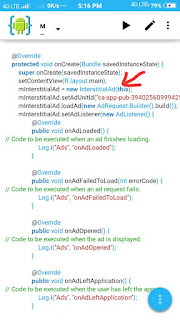
Set Up adListener
mInterstitialAd.setAdListener(new AdListener() {
@Override
public void onAdLoaded() {
// Code to be executed when an ad finishes loading.
Log.i("Ads", "onAdLoaded");
}
@Override
public void onAdFailedToLoad(int errorCode) {
// Code to be executed when an ad request fails.
Log.i("Ads", "onAdFailedToLoad");
}
@Override
public void onAdOpened() {
// Code to be executed when the ad is displayed.
Log.i("Ads", "onAdOpened");
}
@Override
public void onAdLeftApplication() {
// Code to be executed when the user has left the app.
Log.i("Ads", "onAdLeftApplication");
}
@Override
public void onAdClosed() {
// Code to be executed when when the interstitial ad is closed.
Log.i("Ads", "onAdClosed");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
}
});
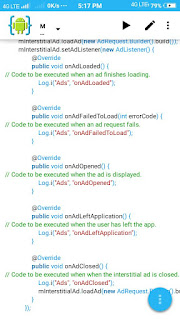
Use this code to display Ads
if (mInterstitialAd.isLoaded()) {
mInterstitialAd.show();
} else {
Log.d("TAG", "The interstitial wasn't loaded yet.");
}

import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.AdRequest;
import com.google.android.gms.ads.InterstitialAd;
import com.google.android.gms.ads.AdListener;
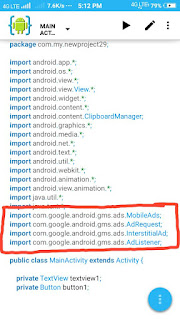
private InterstitialAd mInterstitialAd;
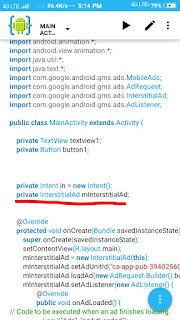
mInterstitialAd = new InterstitialAd(this);
mInterstitialAd.setAdUnitId("ca-app-pub-3940256099942544/1033173712");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
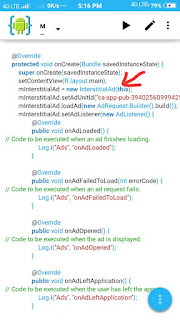
Set Up adListener
mInterstitialAd.setAdListener(new AdListener() {
@Override
public void onAdLoaded() {
// Code to be executed when an ad finishes loading.
Log.i("Ads", "onAdLoaded");
}
@Override
public void onAdFailedToLoad(int errorCode) {
// Code to be executed when an ad request fails.
Log.i("Ads", "onAdFailedToLoad");
}
@Override
public void onAdOpened() {
// Code to be executed when the ad is displayed.
Log.i("Ads", "onAdOpened");
}
@Override
public void onAdLeftApplication() {
// Code to be executed when the user has left the app.
Log.i("Ads", "onAdLeftApplication");
}
@Override
public void onAdClosed() {
// Code to be executed when when the interstitial ad is closed.
Log.i("Ads", "onAdClosed");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
}
});
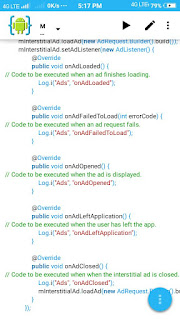
Use this code to display Ads
if (mInterstitialAd.isLoaded()) {
mInterstitialAd.show();
} else {
Log.d("TAG", "The interstitial wasn't loaded yet.");
}

Video Link
If you have any doubts you can comment below
Hello sir how to screen up and down in sketchuaire
ReplyDelete